Memberstack is currently LIVE on Product Hunt! Click here to Join the Discussion →
Find anything
⌘ + K
Ask the AI NEW
Search Help Center
Ask the Community
Message Support
Slack Channel
Product Wishlist
Report a Bug
Twitter - @Memberstack
System Status
Terms & Privacy PoliciesSomething not working as expected?
We would really appreciate if you could let us know what the issue is! If you want us to get back to you about it, you can leave your email too.
Thank you! Your feedback is much appreciated 💙
Oops! Something went wrong while submitting the form.
MemberScripts
An attribute-based solution to add features to your Webflow site.
Simply copy some code, add some attributes, and you're done.
Thank you! Your submission has been received!
Oops! Something went wrong while submitting the form.
Need help with MemberScripts?
All Memberstack customers can ask for assistance in the 2.0 Slack. Please note that these are not official features and support cannot be guaranteed.
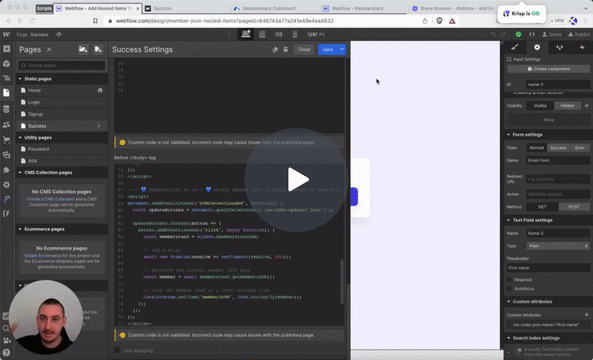
JSON
#4 - Update Member JSON In Local Storage On Click
Add this attribute to any button/element which you want to trigger a JSON update after a short delay.
v0.1
View script
<!-- 💙 MEMBERSCRIPT #4 v0.1 💙 UPDATE MEMBER JSON IN LOCAL STORAGE ON BUTTON CLICK -->
<script>
document.addEventListener("DOMContentLoaded", function() {
const updateButtons = document.querySelectorAll('[ms-code-update="json"]');
updateButtons.forEach(button => {
button.addEventListener("click", async function() {
const memberstack = window.$memberstackDom;
// Add a delay
await new Promise(resolve => setTimeout(resolve, 500));
// Retrieve the current member JSON data
const member = await memberstack.getMemberJSON();
// Save the member JSON as a local storage item
localStorage.setItem("memberJSON", JSON.stringify(member));
});
});
});
</script>
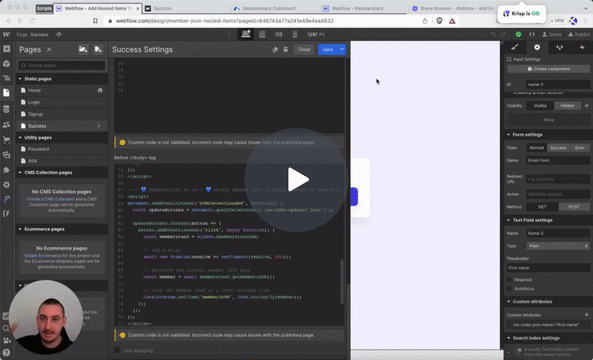
JSON
#3 - Save Member JSON To Local Storage On Page Load
Create a localstorage object containing the logged in Member JSON on page load
v0.1
View script
<!-- 💙 MEMBERSCRIPT #3 v0.1 💙 SAVE JSON TO LOCALSTORAGE ON PAGE LOAD -->
<script>
document.addEventListener("DOMContentLoaded", async function() {
const memberstack = window.$memberstackDom;
// Retrieve the current member JSON data
const member = await memberstack.getMemberJSON();
// Save the member JSON as a local storage item
localStorage.setItem("memberJSON", JSON.stringify(member));
});
</script>
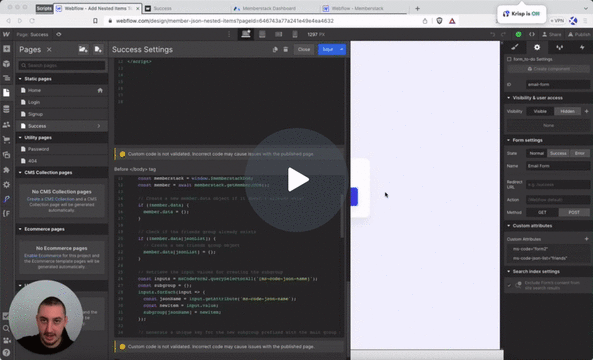
JSON
#2 - Add Item Groups To Member JSON
Allow members to add nested items/groups to their Member JSON.
v0.1
View script
<!-- 💙 MEMBERSCRIPT #2 v0.1 💙 ADD ITEM GROUPS TO MEMBER JSON -->
<script>
document.addEventListener("DOMContentLoaded", function() {
const msCodeForm2 = document.querySelector('[ms-code="form2"]');
const jsonList = msCodeForm2.getAttribute("ms-code-json-list");
msCodeForm2.addEventListener('submit', async function(event) {
event.preventDefault(); // Prevent the default form submission
// Retrieve the current member JSON data
const memberstack = window.$memberstackDom;
const member = await memberstack.getMemberJSON();
// Create a new member.data object if it doesn't already exist
if (!member.data) {
member.data = {};
}
// Check if the friends group already exists
if (!member.data[jsonList]) {
// Create a new friends group object
member.data[jsonList] = {};
}
// Retrieve the input values for creating the subgroup
const inputs = msCodeForm2.querySelectorAll('[ms-code-json-name]');
const subgroup = {};
inputs.forEach(input => {
const jsonName = input.getAttribute('ms-code-json-name');
const newItem = input.value;
subgroup[jsonName] = newItem;
});
// Generate a unique key for the new subgroup prefixed with the main group name
const timestamp = Date.now();
const subgroupKey = `${jsonList}-${timestamp}`;
// Create the new subgroup within the friends group
member.data[jsonList][subgroupKey] = subgroup;
// Update the member JSON with the new data
await memberstack.updateMemberJSON({
json: member.data
});
// Log a message to the console
console.log(`New subgroup with key ${subgroupKey} has been created within ${jsonList} group.`);
// Reset the input values
inputs.forEach(input => {
input.value = "";
});
});
});
</script>
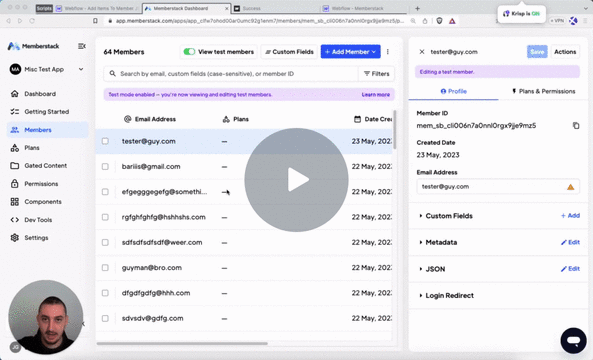
JSON
#1 - Add Items To Member JSON
Allow members to save simple items to their JSON without writing any code.
v0.1
View script
<!-- 💙 MEMBERSCRIPT #1 v0.1 💙 ADD INDIVIDUAL ITEMS TO MEMBER JSON -->
<script>
document.addEventListener("DOMContentLoaded", function() {
const forms = document.querySelectorAll('[ms-code="form1"]');
forms.forEach(form => {
const jsonType = form.getAttribute("ms-code-json-type");
const jsonList = form.getAttribute("ms-code-json-list");
form.addEventListener('submit', async function(event) {
event.preventDefault(); // Prevent the default form submission
// Retrieve the current member JSON data
const memberstack = window.$memberstackDom;
const member = await memberstack.getMemberJSON();
// Create a new member.data object if it doesn't already exist
if (!member.data) {
member.data = {};
}
if (jsonType === "group") {
// Check if the group already exists
if (!member.data[jsonList]) {
// Create a new group object
member.data[jsonList] = {};
}
// Iterate over the inputs with ms-code-json-name attribute
const inputs = form.querySelectorAll('[ms-code-json-name]');
inputs.forEach(input => {
const jsonName = input.getAttribute('ms-code-json-name');
const newItem = input.value;
member.data[jsonList][jsonName] = newItem;
});
// Log a message to the console for group type
console.log(`Item(s) have been added to member JSON with group key ${jsonList}.`);
} else if (jsonType === "array") {
// Check if the array already exists
if (!member.data[jsonList]) {
// Create a new array
member.data[jsonList] = [];
}
// Retrieve the input values for the array type
const inputs = form.querySelectorAll('[ms-code-json-name]');
inputs.forEach(input => {
const jsonName = input.getAttribute('ms-code-json-name');
const newItem = input.value;
member.data[jsonList].push(newItem);
});
// Log a message to the console for array type
console.log(`Item(s) have been added to member JSON with array key ${jsonList}.`);
} else {
// Retrieve the input value and key for the basic item type
const input = form.querySelector('[ms-code-json-name]');
const jsonName = input.getAttribute('ms-code-json-name');
const newItem = input.value;
member.data[jsonName] = newItem;
// Log a message to the console for basic item type
console.log(`Item ${newItem} has been added to member JSON with key ${jsonName}.`);
}
// Update the member JSON with the new data
await memberstack.updateMemberJSON({
json: member.data
});
// Reset the input values
const inputs = form.querySelectorAll('[ms-code-json-name]');
inputs.forEach(input => {
input.value = "";
});
});
});
});
</script>
We couldn't find any scripts for that search... please try again.
Try Memberstack for free
100% free, unlimited trial — upgrade only when you're ready to launch. No credit card required.
Product
Full Feature ListUser AccountsGated ContentSecure PaymentsAPI & IntegrationsCreate a new account2.0 Log in1.0 Log inPricingLanguage
Privacy PolicyTerms of ServiceCookie PolicySecurity Policy
© Memberstack Inc. 2018 – 2024. All rights reserved.