This script redirects inactive users to a "Session Expired" page after a certain period of inactivity. A countdown time is displayed on the page and resets with page activity.
Need help with this MemberScript?
All Memberstack customers can ask for assistance in the 2.0 Slack. Please note that these are not official features and support cannot be guaranteed.
Add this code before the closing </body> tag any page which needs a countdown timer.
Add this code to your /expired page before the closing </body> tag.
Countdown Timer
v0.2 - Attribute value sets timer
This script will now set the logout timer based on the content of the [ms-code-logout-timer] element when the page loads. Please note that the content of this element should be in the format MM:SS.
Here's a simple summary of what this script does:
1. When the webpage first loads, it looks for an HTML element on the page that has the attribute `ms-code-logout-timer`. It expects the content of this element to be a time like "10:00" (for 10 minutes) or "00:30" (for 30 seconds).
2. It then starts a countdown from the specified time. This countdown is displayed on the page by updating the content of the `ms-code-logout-timer` element every second.
3. The script also keeps an eye on any activity on the page, such as moving the mouse, pressing a key, or touching the screen (on a touch-enabled device). If it detects any of these activities, it resets the countdown back to its original starting value.
4. If the countdown ever reaches zero, it will automatically redirect the user to a page with the URL "/expired". After the redirection, the countdown restarts from the initial time.
5. Lastly, any member who lands on the /expired page will be logged out automatically.
Creating the Make.com Scenario
1. Download the JSON blueprint below to get stated.
2. Navigate to Make.com and Create a New Scenario...
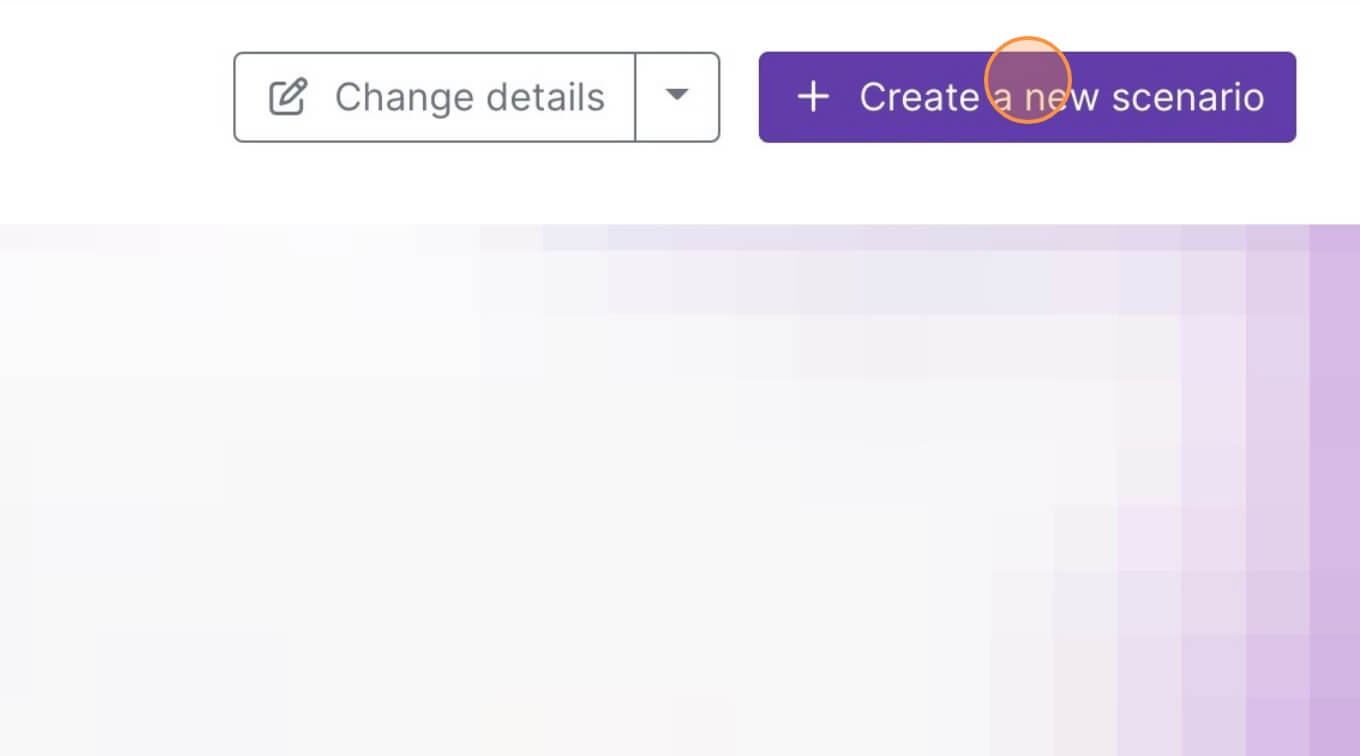
3. Click the small box with 3 dots and then Import Blueprint...
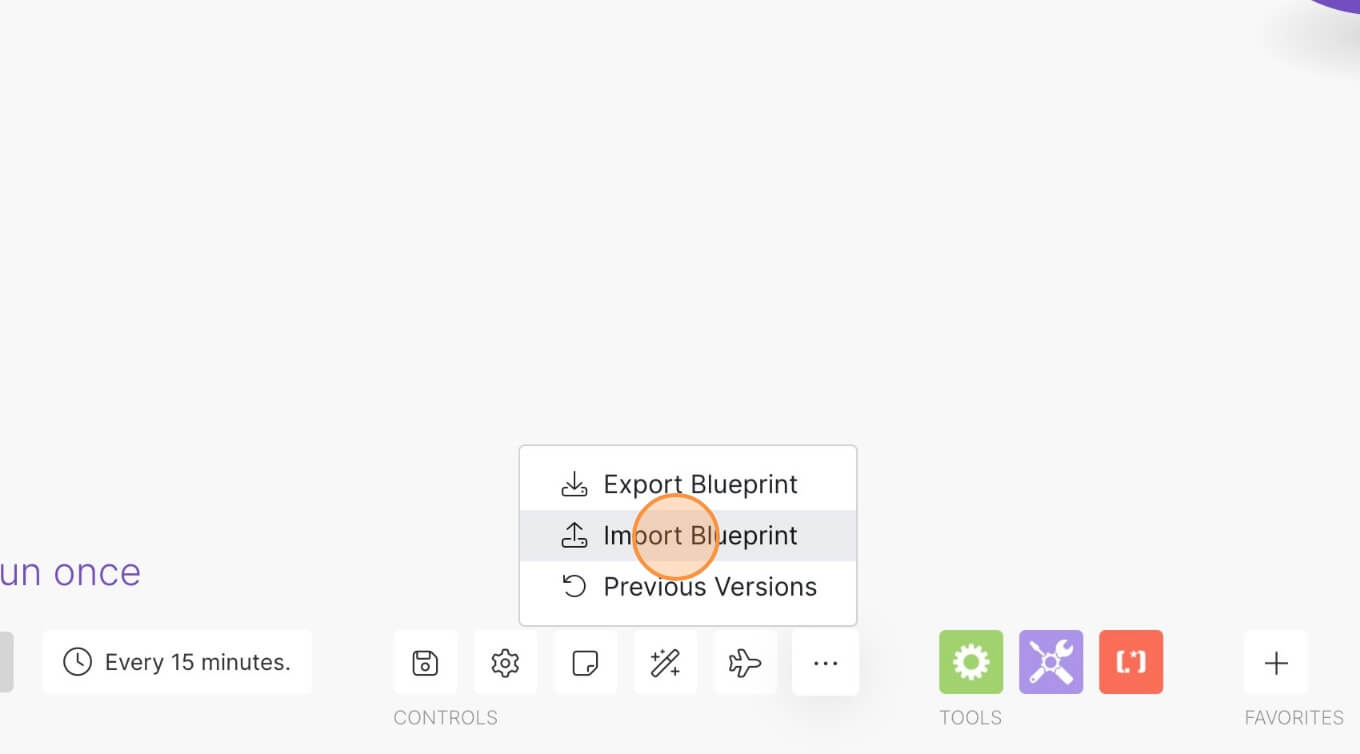
4. Upload your file and voila! You're ready to link your own accounts.
How to Automatically Logout Inactive Users
Memberscripts needed
https://www.memberstack.com/scripts/end-session-after-x-minutes-of-inactivity
Tutorial
Cloneable
https://webflow.com/made-in-webflow/website/automatic-logout
Why/When would need to Automatically Logout Inactive Users?
- Enhanced Security: It prevents unauthorized access to user accounts, especially in shared or public computer scenarios.
- Data Protection: Protects sensitive user data from being exposed.
- Compliance with Policies: Meets certain regulatory and compliance standards that require automatic logout for inactivity.
How to Automatically Logout Inactive Users
Automatic logouts end a user’s session by logging them out after a period of inactivity. Sites include them to improve the security and integrity of user sessions. Limiting the length of inactive sessions can help protect sensitive information and prevent unauthorized account access.
Implementing Automatic Logout in Webflow
We will use a MemberScript that provides all the necessary custom code to add this feature. The script tracks how long a user has been inactive and ends their session if they’ve been inactive for longer than the preset duration.
We assume you’ve already set up Memberstack on your site and allow users to log in to an account managed using Memberstack. If you’re not there yet, don’t worry! You can get started here and come back when you’re set up.
1. Decide a Maximum Length for Inactive Sessions
Your users will have their own patterns when interacting with your site. If you have data on how long their average periods of inactivity are, you can use it to inform your choice of timeout. Having a corporate or regulatory requirement also makes the choice of timeout easier. If you don’t have data or specific requirements, you can make an educated guess here and adjust later if needed.
2. Add Automatic Logout Functionality to Your Webflow Site
We’ll follow the tutorial for MemberScript #16 - End Session After X Minutes of Inactivity to add an automatic logout. After adding the script, your site should log out inactive users after the default time limit has elapsed. Update the time limit using the appropriate maximum inactive session length you determined earlier and give it a manual test to ensure you’ve configured the script correctly.
3. Monitor
Setting the timeout for inactive sessions too low can annoy users and make them more likely to churn. Watch for user feedback related to the automatic logouts to learn more about how well you’ve chosen the timeout length. You might also consider setting up analytics to collect data on the percentage of user sessions automatically logged out and the average session length.
Conclusion
Automatically logging out inactive users is a great way to protect sensitive user data and secure user accounts. By choosing your maximum inactive session length appropriately and using our MemberScript to set up the automatic logout, you can improve the security of your Webflow site.
You can explore the example used in MemberScript #16’s tutorial through this clonable. It includes a page that can only be accessed by members who are logged in, and another that displays after a user has been logged out.
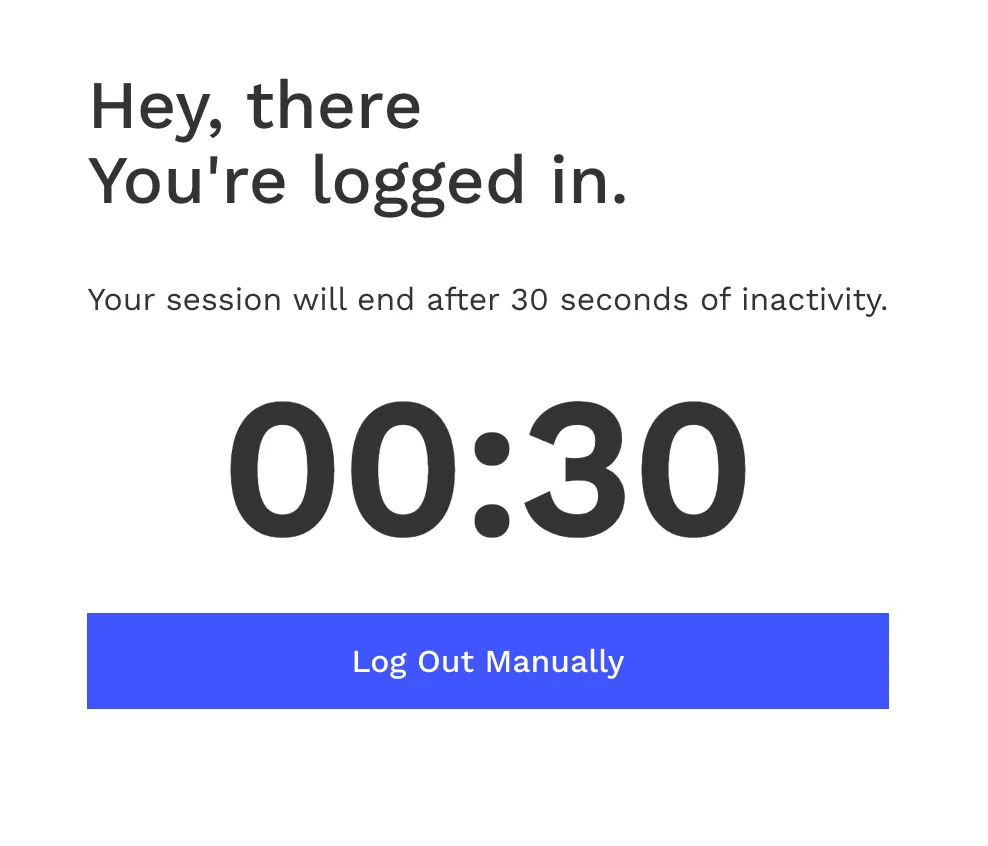
Take me to the Script
https://www.memberstack.com/scripts/end-session-after-x-minutes-of-inactivity