Commenting for Webflow is LIVE on Product Hunt! Get 50% off for 12 months →
Competition: Build a SaaS app between May 29 - June 14 and get a chance to win cash prizes, free plans & more!
#9 - Real, User Based Countdown v0.1
Dynamically set a per-user countdown time and then hide elements when time is up.
View demo
<!-- 💙 MEMBERSCRIPT #9 v0.1 💙 COUNTDOWN BY USER -->
<script>
// Step 1: Get the current date and time
const currentDate = new Date();
// Step 2: Calculate the new date and time based on the attribute values
const addTime = (date, unit, value) => {
const newDate = new Date(date);
switch (unit) {
case 'hour':
newDate.setHours(newDate.getHours() + value);
break;
case 'minute':
newDate.setMinutes(newDate.getMinutes() + value);
break;
case 'second':
newDate.setSeconds(newDate.getSeconds() + value);
break;
case 'millisecond':
newDate.setMilliseconds(newDate.getMilliseconds() + value);
break;
}
return newDate;
};
// Retrieve attribute values and calculate the new date and time
const hourAttr = document.querySelector('[ms-code-time-hour]');
const minuteAttr = document.querySelector('[ms-code-time-minute]');
const secondAttr = document.querySelector('[ms-code-time-second]');
const millisecondAttr = document.querySelector('[ms-code-time-millisecond]');
const countdownDateTime = localStorage.getItem('countdownDateTime');
let newDate;
if (countdownDateTime) {
newDate = new Date(countdownDateTime);
} else {
newDate = currentDate;
if (hourAttr.hasAttribute('ms-code-time-hour')) {
const hours = parseInt(hourAttr.getAttribute('ms-code-time-hour'));
newDate = addTime(newDate, 'hour', isNaN(hours) ? 0 : hours);
}
if (minuteAttr.hasAttribute('ms-code-time-minute')) {
const minutes = parseInt(minuteAttr.getAttribute('ms-code-time-minute'));
newDate = addTime(newDate, 'minute', isNaN(minutes) ? 0 : minutes);
}
if (secondAttr.hasAttribute('ms-code-time-second')) {
const seconds = parseInt(secondAttr.getAttribute('ms-code-time-second'));
newDate = addTime(newDate, 'second', isNaN(seconds) ? 0 : seconds);
}
if (millisecondAttr.hasAttribute('ms-code-time-millisecond')) {
const milliseconds = parseInt(millisecondAttr.getAttribute('ms-code-time-millisecond'));
newDate = addTime(newDate, 'millisecond', isNaN(milliseconds) ? 0 : milliseconds);
}
localStorage.setItem('countdownDateTime', newDate);
}
// Step 4: Update the text of the elements to show the continuous countdown
const countdownElements = [hourAttr, minuteAttr, secondAttr, millisecondAttr];
const updateCountdown = () => {
const currentTime = new Date();
const timeDifference = newDate - currentTime;
if (timeDifference > 0) {
const timeParts = [
Math.floor(timeDifference / (1000 * 60 * 60)) % 24, // Hours
Math.floor(timeDifference / (1000 * 60)) % 60, // Minutes
Math.floor(timeDifference / 1000) % 60, // Seconds
Math.floor(timeDifference) % 1000, // Milliseconds
];
// Update the text of the elements with the countdown values
countdownElements.forEach((element, index) => {
const timeValue = timeParts[index];
if (index === 3) {
element.innerText = timeValue.toString().padStart(2, '0').slice(0, 2); // Display only two digits for milliseconds
} else {
element.innerText = timeValue < 10 ? `0${timeValue}` : timeValue;
}
});
// Update the countdown every 10 milliseconds
setTimeout(updateCountdown, 10);
} else {
// Countdown has reached zero or has passed
countdownElements.forEach((element) => {
element.innerText = '00';
});
// Remove elements with ms-code-countdown="hide-on-end" attribute
const hideOnEndElements = document.querySelectorAll('[ms-code-countdown="hide-on-end"]');
hideOnEndElements.forEach((element) => {
element.remove();
});
// Optionally, you can perform additional actions or display a message when the countdown ends
}
};
// Start the countdown
updateCountdown();
</script>
Creating the Make.com Scenario
1. Download the JSON blueprint below to get stated.
2. Navigate to Make.com and Create a New Scenario...

3. Click the small box with 3 dots and then Import Blueprint...

4. Upload your file and voila! You're ready to link your own accounts.
Need help with this MemberScript?
All Memberstack customers can ask for assistance in the 2.0 Slack. Please note that these are not official features and support cannot be guaranteed.
Join the 2.0 SlackVersion notes
Attributes
Description
Attribute
No items found.
Guides / Tutorials
Tutorial
What is Memberstack?
Auth & payments for Webflow sites
Add logins, subscriptions, gated content, and more to your Webflow site - easy, and fully customizable.
Learn more
.webp)
"We've been using Memberstack for a long time, and it has helped us achieve things we would have never thought possible using Webflow. It's allowed us to build platforms with great depth and functionality and the team behind it has always been super helpful and receptive to feedback"

Jamie Debnam
39 Digital
"Been building a membership site with Memberstack and Jetboost for a client. Feels like magic building with these tools. As someone who’s worked in an agency where some of these apps were coded from scratch, I finally get the hype now. This is a lot faster and a lot cheaper."

Félix Meens
Webflix Studio
"One of the best products to start a membership site - I like the ease of use of Memberstack. I was able to my membership site up and running within a day. Doesn't get easier than that. Also provides the functionality I need to make the user experience more custom."

Eric McQuesten
Health Tech Nerds
Off World Depot
"My business wouldn't be what it is without Memberstack. If you think $30/month is expensive, try hiring a developer to integrate custom recommendations into your site for that price. Incredibly flexible set of tools for those willing to put in some minimal efforts to watch their well put together documentation."

Riley Brown
Off World Depot

"The Slack community is one of the most active I've seen and fellow customers are willing to jump in to answer questions and offer solutions. I've done in-depth evaluations of alternative tools and we always come back to Memberstack - save yourself the time and give it a shot."
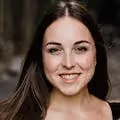
Abbey Burtis
Health Tech Nerds
Slack
Need help with this MemberScript? Join our Slack community!
Join the Memberstack community Slack and ask away! Expect a prompt reply from a team member, a Memberstack expert, or a fellow community member.
Join our SlackTry Memberstack for free
100% free, unlimited trial — upgrade only when you're ready to launch. No credit card required.
Product
Full Feature ListUser AccountsGated ContentSecure PaymentsAPI & IntegrationsMemberstack & WebflowMemberstack & WordPressCreate a new account2.0 Log in1.0 Log inPricingLanguage
Privacy PolicyTerms of ServiceCookie PolicySecurity Policy
© Memberstack Inc. 2018 – 2025. All rights reserved.